Quickstart with sample app
To demonstrate the capabilities of SmartBridge Windows SDK, we have provided a Windows Sample App here.
This guide provides an overview of how to build and run the sample app (optional feature) that we have provided using our Windows SDK.
Note:
This guide provides some basic features only. For full feature code samples, please refer here.
Prerequisites
- Visual Studio 2019 or later
- Windows 10 or later
- Valid SDK credentials (SB Client ID, SB Secret, oAuth URL, API Base URL, and Callback URL)
- Provision online terminal
Note:
You need to be a system administrator to perform this guide for the first time.
Step 1: Create and configure a new project
- Open Visual Studio > Create a new project.
- On the Create a new project window, enter or type "Windows Forms" in the search box.
- Select Dekstop from the Project type list.
- On the Configure your new project, enter Project Name, Location, and select the Framework.
- Click Create.
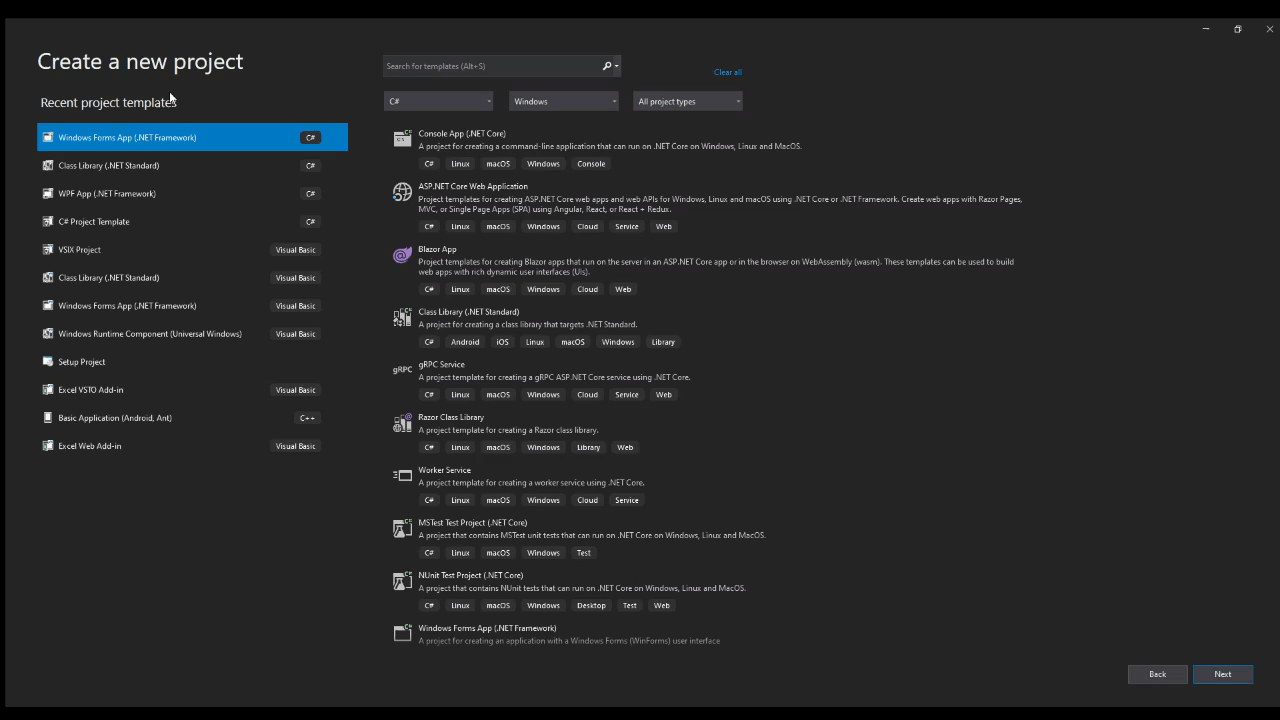
Step 2: Obtain access credentials
- Create another form for login oAuth.
- Under Toolbox, click WebBrowser.
- Under Properties, sets Modifier to be "Public".
- Double-click the form.
- Under
private void webBrowser1_DocumentCompleted(object sender, WebBrowserDocumentCompletedEventArgs e)
, insert the following code:
if (webBrowser1.Url.ToString().Contains("?code="))
{
// we have the code needed for the access token request
this.Tag = webBrowser1.Url.ToString().Substring(webBrowser1.Url.ToString().IndexOf("?code=") + 6);
this.DialogResult = DialogResult.OK;
}
- Save the file and close the login form.
Step 3: Create the forms
In this sample app, we will have three main functions:
- Credentials: to store the SB ClientID, SB Secret, ISV Callback URL, SB Acc Token, and SB Refresh Token.
- Merchant: to log in, link the terminal, and get merchants terminal.
- Transactions: to make a transaction based on the amount and currency and check transaction status.
To build the above functions, simply follow the instructions below:
- Under Toolbox, select All Windows Forms > TabControl and create three tabs for Credentials, Merchant, and Transactions by changing the Text under Properties.
Step 4: Download the NuGet Packages
- Go to Tools > Options.
- Under NuGet Package Manager, click Package Sources.
- Click + to add a new source.
- Set Name and Source.
Note:
Please don't forget to remember the directory that you set to store this package.
- Click OK.
- Download the NuGet Package from Github and put it in your new NuGet Source Directory.
- Under Solution Explorer, right-click the project name and click Manage NuGet Packages.
- Click Browse and My Sources from Package source drop-down menu.
- Click RMS-SB v1 and Install.
Step 5: Develop the UI
- To create a button, under Toolbox, select All Windows Forms > Button.
- To create a label, under Toolbox, select All Windows Forms > Label.
- To create a textbox, under Toolbox, select All Windows Forms > TextBox.
- To create a listview, under Toolbox, select All Windows Forms > ListView.
Note:
Repeat the above steps as needed to create the similar UI that is shown in the GIF below.
- In this example, we will have SB Client ID, SB Secret, ISV Callback Url, SB Acc Token, and SB Refresh Token in Credentials tab.
- In the Merchant tab, create a Login button, a Link Terminal button, a Get Merchants Terminals button, a ListView, and a textbox similar to what is shown in GIF below.
- In the Transactions tab, create a Make Transaction button, a check Transaction status button, an Amount textbox, and a Currency textbox, similar to what is shown in GIF below.
Note:
Please make sure that you rename the button to easily identify each button function. To rename the button for the UI, you need to edit the Text row under the Properties. To rename the button function for the backend code, you need to edit the (Name) row under the Properties.
Step 6: Setup the backend
- To manage a collection of objects, you need to select a textbox and go (ApplicationSettings) > (PropertyBinding) > click the three dots icon under Properties.
Note:
Please ensure that you implement the step above in all of your textboxes.
- Click the Merchant tab and double-click the login button.
- Under
using System.Threading.Tasks;
, insert the following code:
Note:
Please ensure all the codes that you added in the instruction below are within the bracket {} of each function.
using System.Windows.IO;
using Newtonsoft.Json.Linq;
- To make the login button works, insert the following code before the
private void cmdGetAccessToken_Click(object sender, EventArgs e)
:
Note
This void function name represents the button function name. Each button function name represents the name that you set up in step 5 under Properties and (Name) row. For example, we set the login button function name to be cmdGetAccessToken.
private void IEbrowserFix()
{
try
{
Microsoft.Win32.RegistryKey regDM;
bool is64 = Environment.Is64BitOperatingSystem;
string KeyPath = "";
if (is64)
{
KeyPath = @"SOFTWARE\Wow6432Node\Microsoft\Internet Explorer\MAIN\FeatureControl\FEATURE_BROWSER_EMULATION";
}
else
{
KeyPath = @"SOFTWARE\Microsoft\Internet Explorer\MAIN\FeatureControl\FEATURE_BROWSER_EMULATION";
}
regDM = Microsoft.Win32.Registry.LocalMachine.OpenSubKey(KeyPath, false);
if (regDM is null)
{
regDM = Microsoft.Win32.Registry.LocalMachine.CreateSubKey(KeyPath);
}
Microsoft.Win32.RegistryKey Sleutel;
if (regDM is object)
{
string location = Environment.GetCommandLineArgs()[0];
string appName = Path.GetFileName(location);
Sleutel = (Microsoft.Win32.RegistryKey)regDM.GetValue(appName);
if (Sleutel is null)
{
// Sleutel onbekend
regDM = Microsoft.Win32.Registry.LocalMachine.OpenSubKey(KeyPath, true);
Sleutel = Microsoft.Win32.Registry.LocalMachine.CreateSubKey(KeyPath, Microsoft.Win32.RegistryKeyPermissionCheck.ReadWriteSubTree);
// What OS are we using
var OsVersion = Environment.OSVersion.Version;
if (OsVersion.Major == 6 & OsVersion.Minor == 1)
{
// WIN 7
Sleutel.SetValue(appName, 9000, Microsoft.Win32.RegistryValueKind.DWord);
}
else if (OsVersion.Major == 6 & OsVersion.Minor == 2)
{
// WIN 8 and above
Sleutel.SetValue(appName, 10000, Microsoft.Win32.RegistryValueKind.DWord);
}
else if (OsVersion.Major == 5 & OsVersion.Minor == 1)
{
// WIN xp
Sleutel.SetValue(appName, 8000, Microsoft.Win32.RegistryValueKind.DWord);
}
Sleutel.Close();
}
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message + "\r\nYou need to run as Administrator to alter the registry");
}
}
- Under
private void cmdGetAccessToken_Click(object sender, EventArgs e)
, insert the following code:
{
//If you receive script errors on login then call this
// IEbrowserFix();
var rms = new SmartBridge.Api(txtClientID.Text, txtSecret.Text, txtReturnUrl.Text,txtBaseUrl.Text, txtoAuthUrl.Text);
Properties.Settings.Default.Save();
string url = rms.getoAuthUrl();
var frm = new frmlogin();
frm.webBrowser1.Navigate(url);
frm.ShowDialog();
string code = (string)frm.Tag;
frm.Close();
frm = default;
if (string.IsNullOrEmpty(code))
return;
try
{
JObject resp = (JObject)rms.GetToken(code);
txtaccess.Text = (string)resp["access_token"];
txtRefresh.Text = (string)resp["refresh_token"];
MessageBox.Show("Login Successfull");
Properties.Settings.Default.Save();
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
Note:
Please ensure that the name that you set up under Properties and Text row when you create new textbox matches with the function within the backend code. For example, in this sample app, we set the Text row value of the Client ID textbox to be txtClientID. Thus, in the code, we put rms.setKey(txtClientID.Text);
- Go back to Merchant tab and double-click the Link Terminal button.
- Under
private void cmdSetTerminal_Click(object sender, EventArgs e)
, insert the following code:
{
try
{
var rms = new SmartBridge.Api(txtClientID.Text, txtSecret.Text, txtReturnUrl.Text, txtBaseUrl.Text, txtoAuthUrl.Text);
rms.setToken(txtaccess.Text);
rms.setRefreshToken(txtRefresh.Text);
rms.SetActiveTerminal(txtTerminalId.Text);
MessageBox.Show("The terminal has been set");
Properties.Settings.Default.Save();
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
- Go back to Merchant tab and double-click the Get Merchants Terminals button.
- Under
private void cmdGetTerminalList_Click(object sender, EventArgs e)
, insert the following code:
{
var rms = new SmartBridge.Api(txtClientID.Text, txtSecret.Text, txtReturnUrl.Text, txtBaseUrl.Text, txtoAuthUrl.Text);
rms.setToken(txtaccess.Text);
rms.setRefreshToken(txtRefresh.Text);
object terminals;
lvTerminals.Items.Clear();
try
{
terminals = rms.GetTerminalList();
foreach (JObject terminal in (IEnumerable)terminals)
{
var li = new ListViewItem();
li.Text = terminal["terminalId"].ToString();
li.SubItems.Add((terminal["terminalName"] is null)? terminal["terminalId"].ToString():terminal["terminalName"].ToString());
li.SubItems.Add(terminal["terminalStatus"].ToString());
lvTerminals.Items.Add(li);
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
- Click the Transactions tab and double-click the Make Transaction button.
- Under
private void CmdMakeTransaction_Click(object sender, EventArgs e)
, insert the following code:
{
var rms = new SmartBridge.Api(txtClientID.Text, txtSecret.Text, txtReturnUrl.Text, txtBaseUrl.Text, txtoAuthUrl.Text);
rms.setToken(txtaccess.Text);
rms.setRefreshToken(txtRefresh.Text);
rms.SetActiveTerminal(txtTerminalId.Text);
JObject resp;
try
{
resp =(JObject) rms.CreateTransaction(int.Parse(txtamount.Text), txtCurrency.Text, "SALE");
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
return;
}
string transactionid = resp["transactionid"].ToString();
cmdCheckStatus.Tag = transactionid;
MessageBox.Show("Transaction successfull \r\nCheck Status for approval");
}
- Finally, for the final button, go back to Transactions tab and double-click the check Transaction status button.
- Under
private void cmdCheckStatus_Click(object sender, EventArgs e)
, insert the following code:
{
var rms = new SmartBridge.Api(txtClientID.Text, txtSecret.Text, txtReturnUrl.Text, txtBaseUrl.Text, txtoAuthUrl.Text);
rms.setToken(txtaccess.Text);
rms.setRefreshToken(txtRefresh.Text);
rms.SetActiveTerminal(txtTerminalId.Text);
object resp;
try
{
resp = rms.CheckStatus((string)cmdCheckStatus.Tag);
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
return;
}
lblTransstatus.Text = (resp is null)?"":resp.ToString();
}
- Press F5 to run enter your valid SDK credentials.
π¬ We're here to help!
If you're looking for help, shoot us an email. Please include a description of the issues that you are running into.
Updated over 2 years ago